用 A* Search Algorithm 走迷宮
前言
前一篇文章中,我使用了 Eller’s Algorithm 迷宮生成算法 來生成迷宮,這一篇文章會先介紹 A* Algorithm,並使用 A* Search Algorithm 來攻略迷宮,先偷看一下成果:
A* Search Algorithm
A* 是一種最短路徑算法,它跟 Dijkstra 非常地相似,都會根據某個優先序來選擇下一步要走的路徑,唯一不同的點是 Dijkstra 的優先序只考量了目前「已走的距離」,因此它的搜尋方向會朝四周發散。但我們在搜尋路徑時通常會是有目的地,也就是知道方向的,一般情況下,我們不會想往反方向尋找最短路徑,所以 A* 在 Dijkstra 的基礎上多考慮了未來「預期要走的距離」。
簡單地去理解,「已走的距離」+「預期要走的距離」基本上就會是整趟路的距離,而直接用整趟路的距離來排序優線序,大部分情況下都能幫助我們更快找到最短路徑,這取決於我們對預期的估算是否準確。不過也不用想得太複雜,對於這個預期,通常直接用簡單的距離函式求得就好,如歐幾里德距離或曼哈頓距離。
以下圖來說,橘色是已走的距離,Dijkstra 只會考慮這一段,藍色則是預期要走的距離,橘色+藍色就是 A* 會考慮的範圍。
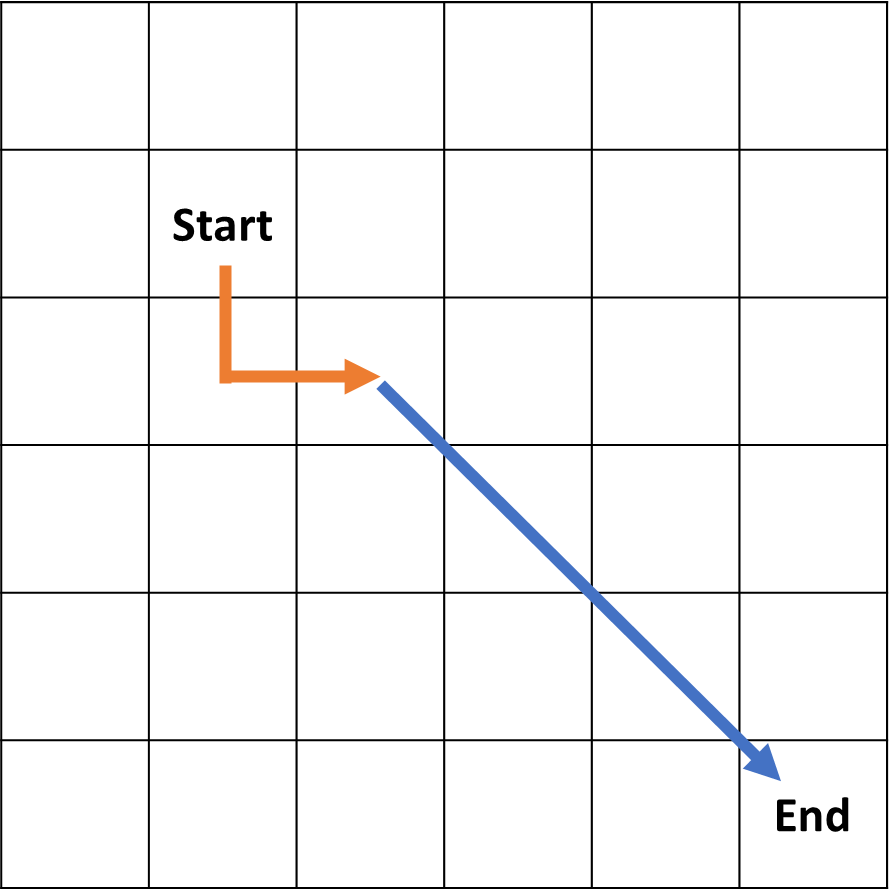
Python 實現
不能說跟 Dijkstra 很像,基本上是一模一樣,只是把 priority queue 裡面內容換成整趟路的距離。
搜索路徑
1 | import heapq |
繪製路徑
1 | import matplotlib.pyplot as plt |
執行結果
1 | height = 20 |
成果如下: